
Co-Founder Innovation Geeks
The Julia Language: Unleashing Speed and Productivity in Scientific Computing
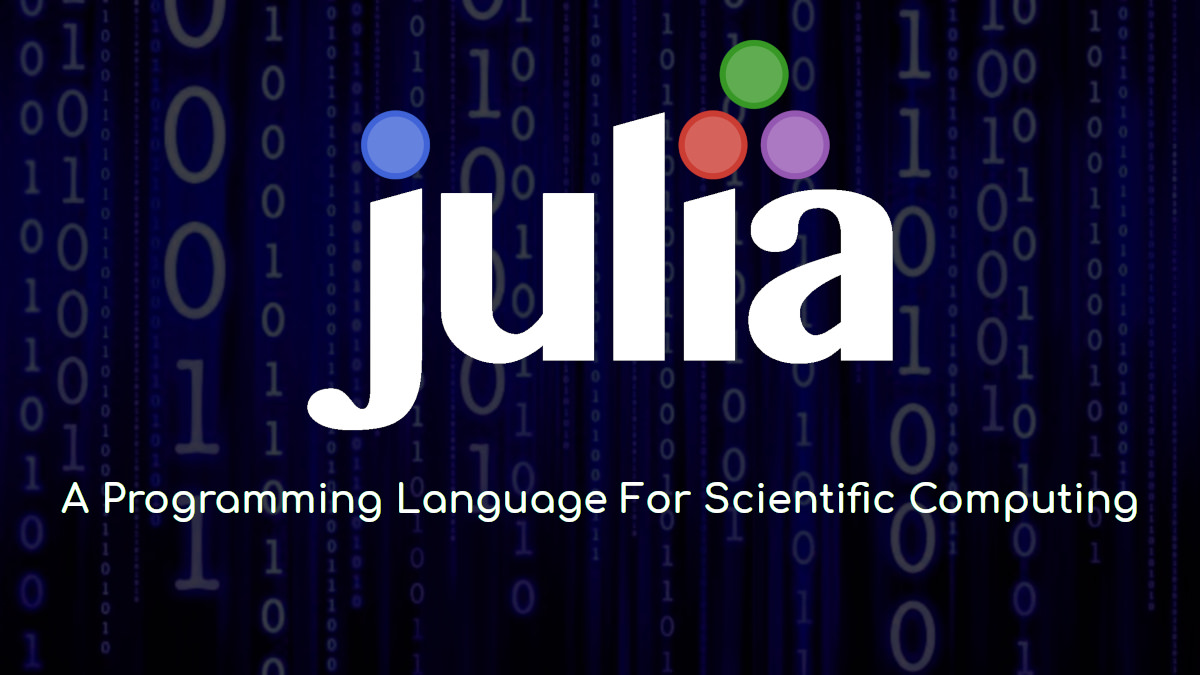
In the ever-evolving landscape of programming languages, a relatively new entrant has been gaining momentum and turning heads, especially in the realm of scientific computing and data analysis. The Julia programming language, first introduced in 2012 by Jeff Bezanson, Stefan Karpinski, Viral B. Shah, and Alan Edelman, has quickly risen to prominence due to its remarkable speed, ease of use, and suitability for high-performance computing tasks. Let's delve deeper into the history of Julia, compare it with Python and R, explore its current applications, provide documentation links, and discuss its significance in the upcoming years.
A Brief History of Julia
The development of Julia was motivated by a desire to create a language that combined the ease of use of high-level languages like Python and R with the performance of low-level languages like C and Fortran. The founders recognized that existing programming languages often required developers to make trade-offs between productivity and performance. Julia was designed to bridge this gap by introducing a dynamic yet high-performance language tailored for scientific and numerical computing.
Julia vs. Python and R: A Comparison
- Speed and Performance
Python and R, while popular in scientific computing and data analysis, are often criticized for their execution speed. Python, due to its interpreted nature, can be slow when performing intensive numerical computations. R, on the other hand, was primarily designed for data analysis and statistics, and its performance can also be suboptimal for complex mathematical calculations.
Julia, with its JIT compiler, shines in this regard. It compiles high-level code into efficient machine code on the fly, resulting in execution speeds comparable to low-level languages like C and Fortran. This makes Julia a go-to choice for computationally demanding tasks. - Syntax and Ease of Use
Python and R are known for their easy-to-understand syntax and large communities, making them accessible to developers of varying skill levels. This is especially beneficial for beginners and those transitioning from other languages.
Julia, while similar in many respects, introduces a few differences in syntax. However, its syntax is intuitive and expressive, making it relatively easy for Python and R users to adapt. Julia's multiple dispatch system and dynamic typing further enhance code clarity and modularity. - Package Ecosystem
Python's extensive package ecosystem, particularly with libraries like NumPy, SciPy, and pandas, has made it a staple in data science and scientific computing. R, with its specialized packages for statistics and data manipulation, also holds a strong position in data analysis.
Julia's package ecosystem, while smaller than Python's and R's, is rapidly growing. Packages likeDataFrames.jl
,Plots.jl
andFlux.jl
are gaining popularity and offering competitive alternatives to their Python and R counterparts. - Interoperability
Both Python and R allow seamless integration with other languages and systems. Python's versatile C and C++ integration and R's ability to call C and Fortran code make them favorable choices when working with legacy codebases.
Julia, known for its excellent interoperability, can directly call C and Fortran functions, simplifying the integration of existing optimized code. Additionally, its Python and R packages facilitate cross-language interaction.
Current Applications of Julia
The versatility of Julia has led to its adoption in various domains:
- 1. Scientific Computing:
Julia's speed and numerical capabilities make it a natural fit for scientific simulations, computational physics, and mathematical modeling. Researchers can quickly prototype and execute complex simulations, accelerating the pace of scientific discovery. - 2. Data Science and Machine Learning:
With packages like Flux.jl and MLJ.jl, Julia is making its mark in the field of machine learning. Its performance advantage is particularly beneficial for training large-scale models on massive datasets. - 3. Finance and Quantitative Analysis:
Julia's speed and ability to handle financial computations efficiently have attracted the attention of the finance industry. Quantitative analysts and researchers can process vast amounts of financial data in real-time, enabling quicker decision-making. - 4. High-Performance Computing (HPC):
Julia's parallel computing capabilities and low-level programming support make it a solid choice for HPC applications. Complex simulations, weather modeling, and other resource-intensive tasks can be executed more efficiently.
Simple Example
Let's take a look at a simple example of implementing a linear regression algorithm using Julia. Linear regression is a fundamental machine learning algorithm used for predicting a continuous target variable based on one or more input features.
using Random, DataFrames, GLM
# Generate some random data for demonstration
Random.seed!(123)
n_samples = 100
X = randn(n_samples, 2) # Features
# Target variable
y = 2 * X[:, 1] - 3 * X[:, 2] + 1 + 0.1 * randn(n_samples)
# Create a DataFrame for easy manipulation
data = DataFrame(X1 = X[:, 1], X2 = X[:, 2], Y = y)
# Fit a linear regression model
model = lm(@formula(Y ~ X1 + X2), data)
# Print model coefficients
println("Model Coefficients:")
println(coef(model))
# Make predictions for new data
new_data = DataFrame(X1 = [0.5, -1.0], X2 = [1.0, 2.0])
predictions = predict(model, new_data)
println("Predictions for new data:")
println(predictions)
In this example, we first generate some random data with two features (`X1`
and X2
) and a target variable (`Y`
). We then create a DataFrame using the DataFrames
package to store and manipulate the data.
Using the `GLM package`
, we fit a linear regression model to the data using the `lm`
function and the provided formula. The `coef`
function is used to print the coefficients of the model, which represent the slopes and intercept.
Finally, we use the trained model to make predictions for new data points with the `predict`
function. The output shows the predicted values based on the linear regression model. Keep in mind that this example uses randomly generated data for demonstration purposes. In practice, you would typically work with real-world datasets for training and evaluating machine learning models.
Learning Resources and Documentation
For those eager to explore the Julia programming language further, here are some essential resources:
- Official Julia Documentation: The official documentation provides a comprehensive guide to the language's syntax, features, and usage. It's an excellent starting point for beginners.
- JuliaAcademy: JuliaAcademy offers interactive courses for learning Julia. From introductory to advanced topics, these courses cater to learners of various skill levels.
- Julia Discourse: This community forum is a hub for discussions, questions, and announcements related to Julia. Engaging with the community can be immensely helpful in gaining insights and solving challenges.
- JuliaHub: JuliaHub provides an environment for hosting, sharing, and discovering Julia packages. It's a valuable resource for exploring the diverse package ecosystem.